
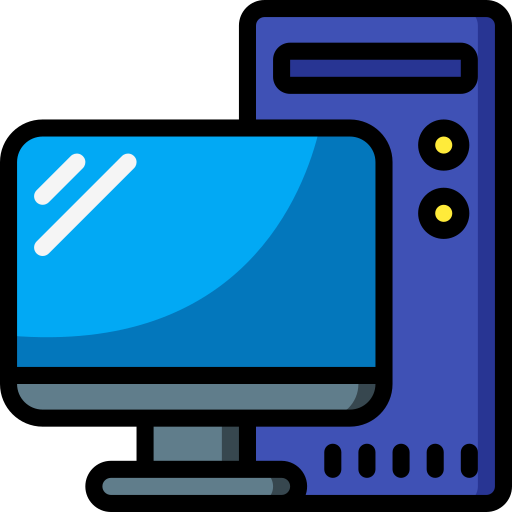
Sure thing - one thing I’ll often do for stuff like this is spin up a VM. You can throw 4x1GiB virtual drives in it and play around with creating and managing a raid using whatever you like. You can try out md, ZFS, and BTRFS without any risk - even unraid.
Another variable to consider as well - different RAID systems have different flexibility for reshaping the RAID. For example - if you wanted to add a disk later, or swap out old drives for new ones to increase space. It’s yet another rabbit hole to go down, but something to keep in mind. When we start talking about 10’s of terrabytes of data you start to lose somewhere to temporarily put it all if you need to recreate your raid to change your raid layout. :-)
It’s really quite simple - but works pretty well. There are 3 components:
Kiosk service
A simple systemd service that starts a kiosk script.
[Unit] Description=Kiosk Wants=graphical.target After=graphical.target [Service] Environment=DISPLAY=:0.0 Environment=XAUTHORITY=/home/pi/.Xauthority Type=simple ExecStart=/bin/bash /home/pi/kiosk.sh Restart=on-abort User=pi Group=pi [Install] WantedBy=graphical.target
Kiosk script
The script in /home/pi/kiosk.sh just starts a web browser in full-screen mode pointed at my home assistant instance:
#!/bin/bash xset s noblank xset s off xset -dpms export DISPLAY=:0.0 echo 0 > /sys/class/backlight/rpi_backlight/bl_power LANDING_PAGE="https://homeassistant.example.com/" unclutter -idle 0.5 -root & /usr/bin/chromium-browser --noerrdialogs --disable-infobars --kiosk $LANDING_PAGE
Display service
I have a very simple python/flask service that runs and exposes an endpoint that lets you turn on/off the display. It’s called by a homeassistant automation for when the motion detector senses or hasn’t sensed movement.
Here’s the python - I have this started from another “kiosk.service” systemd service as well.
#!/usr/bin/env python3 import subprocess from flask import Flask from flask_restful import Api, Resource def turn_off_display(): with(open(backlight_dev, 'w')) as dev: dev.write("1") def turn_on_display(): with(open(backlight_dev, 'w')) as dev: dev.write("0") class DisplayController(Resource): def get(self, state): if state == 'off': turn_off_display() elif state == 'on': turn_on_display() else: return {'message': f'Unknown state {state} - should be off/on'}, 500 return {"message": "Success"} def init(): turn_on_display() if __name__ == "__main__": init() app = Flask(__name__) api = Api(app) api.add_resource(DisplayController, '/display/<string:state>') app.run(debug=False, host='0.0.0.0', port=3000)
You can then have the HA rest action call this with “http://pidisplay:3000/display/on” or off.